Discover the enchanting power of recursion as we unravel the secret to summing natural numbers effortlessly.
Table of Contents
Welcome to our curated collection of blog posts, where we dive into the fascinating realm of programming with a friendly and approachable tone. In this edition, we explore the program that sums up natural numbers using recursion. Recursion is a powerful technique in programming, and understanding how to use it effectively for solving problems is a valuable skill for any programmer.
Understanding Recursion
Before we delve into solving the sum of natural numbers program using recursion, let’s first ensure we have a solid understanding of what recursion actually is. Recursion is a programming concept where a function calls itself as a subroutine, creating a loop of repetitive actions until a specific condition, known as the base case, is met.
Unlock the Power of Recursion: Summing Natural Numbers
Subscribe Today and Dive Deep into the Fascinating World of Recursion!
The concept of recursive functions may sound complex, but let’s break it down into simpler terms. Imagine you have a task to complete, and to accomplish it, you need to perform the same action repeatedly until a certain condition is fulfilled. This is essentially what a recursive function does – it breaks down a problem into smaller, more manageable tasks, and solves each smaller task until the overall problem is solved.
While recursion can be a powerful and elegant solution to certain problems, it’s important to note that it may not always be the most efficient approach. Recursion can lead to intensive use of memory and may be slower compared to iterative methods for solving some problems. Therefore, it’s crucial to weigh the pros and cons of using recursion in each specific scenario.
Step-by-Step Program Implementation
Now that we have a good understanding of recursion, let’s move on to implementing the sum of natural numbers program. We’ll be using a popular programming language like Python for demonstration purposes.
To start, we need to define a recursive function that calculates the sum of natural numbers up to a given input. Let’s name our function “sum_of_naturals” for simplicity.
First, we need to identify the base case – the condition that tells our function when to stop calling itself. In this case, the base case occurs when the input number is 1. When this happens, we know we have reached the end of the recursion and can return 1.
Next, we define the recursive step. In this step, we call our “sum_of_naturals” function with the input number decremented by 1 and add it to the current value of the input number.
Let’s take a look at the recursive function in Python:
“`python def sum_of_naturals(n): if n == 1: return 1 else: return n + sum_of_naturals(n – 1) “`
Now that we have our recursive function implemented, let’s walk through an example to understand how it works. Let’s call our “sum_of_naturals” function with the input number 5:
“`python print(sum_of_naturals(5)) “`
When we call “sum_of_naturals(5)”, the function checks if the input number (5) is equal to 1. Since it’s not, it proceeds to the else statement and returns 5 + “sum_of_naturals(4)”.
The function then repeats the process and checks if “sum_of_naturals(4)” evaluates to the base case. It continues this recursive process until it reaches “sum_of_naturals(1)”, which equals 1 (the base case). Once this base case is met, each intermediate sum is returned, and the recursion starts unwinding.
The final result, when we print the output, will be 15 – the sum of natural numbers up to 5.
Analyzing the Complexity
When dealing with recursion, it’s important to analyze the complexity of our solution. Complexity analysis helps us understand how our program performs in terms of time and space efficiency.

In the case of the sum of natural numbers program implemented using recursion, the time complexity is O(n) and the space complexity is also O(n).
The time complexity of O(n) indicates that the time taken to execute the program increases linearly with the size of the input number. The space complexity of O(n) means that the amount of memory required by the program also increases linearly with the input size.
To compare recursion with an alternative solution, let’s briefly touch on the iterative approach. An iterative solution can achieve the same result as the recursive solution. However, it often requires an explicit loop construct and additional variables to keep track of the sum. Nonetheless, the iterative solution usually has a better performance in terms of memory and time complexity.
Best Practices and Optimization Techniques
While we’ve successfully implemented the sum of natural numbers program using recursion, it’s important to be aware of some potential pitfalls and consider optimization techniques for more complex recursive problems.
One common pitfall to avoid is infinite recursion. If the base case is not properly defined or the recursive step is not modifying the input number correctly, the function may end up calling itself indefinitely, leading to a program crash. Always double-check the base case condition and make sure the input is modified correctly in each recursive call.
When it comes to optimization, one technique is tail recursion optimization. Tail recursion occurs when a recursive function makes its recursive call as the last action in the function, allowing the compiler or interpreter to optimize the recursive process. In some programming languages like Scheme or Erlang, tail recursion optimization is automatically applied. However, mainstream languages like Python may not guarantee tail recursion optimization, so using an iterative approach can often be more efficient.
Another optimization technique is memoization. Memoization involves caching the results of expensive function calls and reusing them when the same function is called again with the same inputs. While this technique may not be applicable to the sum of natural numbers program, it can greatly improve the performance of recursive functions that involve repetitive calculations.
Real-Life Applications
Although the sum of natural numbers program may seem simplistic, the concept of recursion is widely used in various real-life applications. Recursive algorithms are heavily used in mathematics, computer science, and artificial intelligence, among other fields.
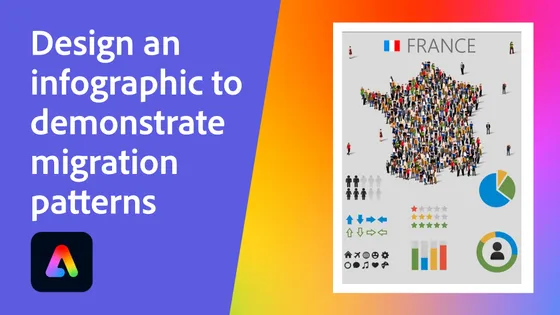
For example, in mathematics, recursion is integral to calculations involving factorials, Fibonacci numbers, and fractals. In computer science, recursive algorithms are commonly used for tree traversal, searching, sorting, and backtracking problems. In artificial intelligence, recursive neural networks model human cognition.
By mastering the sum of natural numbers program and understanding the underlying principles of recursion, you’ll be equipped to tackle more complex problems and create efficient and elegant solutions.
Unlock the Power of Recursion: Summing Natural Numbers
Subscribe Today and Dive Deep into the Fascinating World of Recursion!
Conclusion
In this curated collection, we’ve explored the magic of recursion by implementing the sum of natural numbers program. Recursion is a powerful technique in programming that allows you to break down complex problems into simpler steps. By understanding the base case, defining the recursive step, and considering optimization techniques, you can leverage the power of recursion to develop efficient and elegant solutions.
Remember, practice is key to mastering recursion. Experiment with different recursive problems, analyze their complexity, and explore optimization possibilities. With time and experience, recursion will become an invaluable tool in your programming arsenal.
Happy coding!